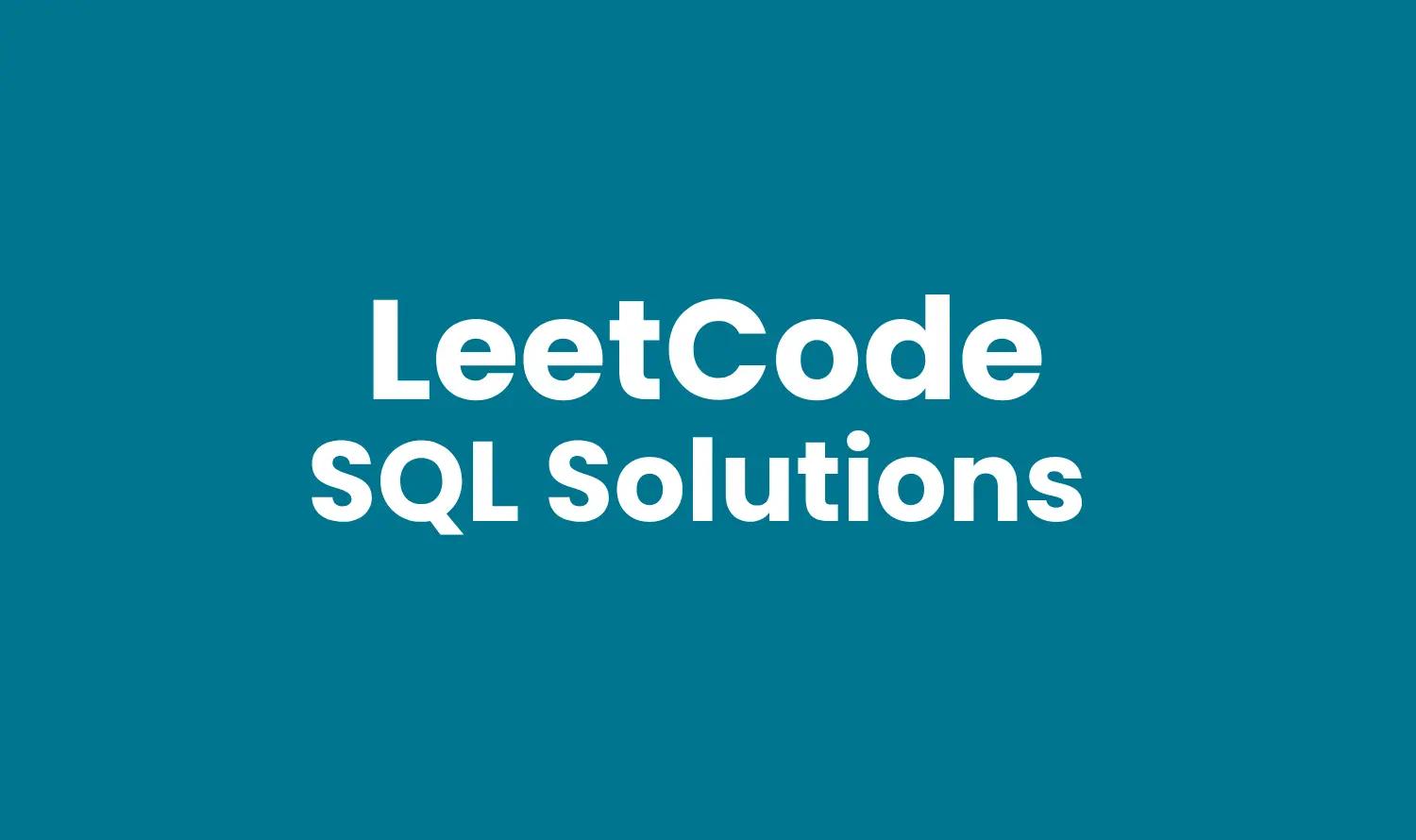
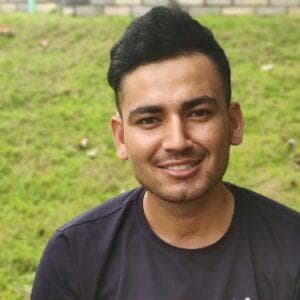
Tilak Khatri
1251. Average Selling Price
When working with databases, a common task is to calculate averages based on certain conditions. In this scenario, we want to calculate the average price of products based on the units sold within specific date ranges. This involves using SQL to join tables, perform calculations, and handle potential pitfalls like integer division and missing data.
Problem Breakdown
1. Tables Involved:
- Prices Table: Contains information about product prices over specific date ranges
- Columns: product_id, start_date, end_date, price
- UnitsSold Table: Contains records of units sold on specific dates.
- Columns: product_id, purchase_date, units
2. Objective:
Calculate the weighted average price for each product, considering only the units sold within the price's date range.
3. Weighted Average Formula:
The weighted average price is calculated as:
1average_price=∑(price×units)∑(units)average_price=∑(units)∑(price×units)
This formula ensures that the average price reflects the actual sales volume.
SQL Query Explanation
To achieve the desired output, we use an SQL query that performs the following steps:
1. Join the Tables:
- Use a LEFT JOIN to combine the Price and UnitsSold tables based on product_id.
- Ensure that the purchase_date from UnitsSold falls between the start_date and end_date from prices.
2. Calculate the Weighted Average:
- Multiply the price by units for each record to get the total sales value.
- Sum these values and divide by the total units sold to get the average price.
- Use the ROUND function to ensure the result is rounded to two decimal places.
3. Handle Edge Cases:
- Use a CASE statement to handle scenarios where no units are sold, preventing division by zero.
Example Query
Here's the SQL query that implements the above logic:
1SELECT
2 t1.product_id,
3 ROUND(
4 CASE
5 WHEN SUM(t2.units) > 0 THEN SUM(CAST(t1.price AS DECIMAL) * t2.units) / SUM(t2.units)
6 ELSE 0
7 END,
8 2) AS average_price
9FROM
10 prices t1
11LEFT JOIN
12 unitssold t2
13ON
14 t1.product_id = t2.product_id
15 AND t2.purchase_date BETWEEN t1.start_date AND t1.end_date
16GROUP BY
17 t1.product_id;
Key Takeaways
- Data Integrity: Ensure that your data types support decimal arithmetic to avoid losing precision.
- SQL Functions: Use ROUND to control the number of decimal places in your results.
- Error Handling: Use CASE statements to handle potential errors like division by zero.
- Join Conditions: Carefully construct your join conditions to ensure that only relevant data is included in calculations.